1) Write a program to read 10 integers in an array. Find the addition of all elements.
#include<stdio.h>
#include<conio.h>
void main()
{
int arr[10],i,total=0;
clrscr();
for(i=0;i<10;i++)
{
printf("Enter an integer: ");
scanf("%d", &arr[i]);
total=total+arr[i];
}
printf("The sum of the array elements is: %d\n", total);
getch();
}
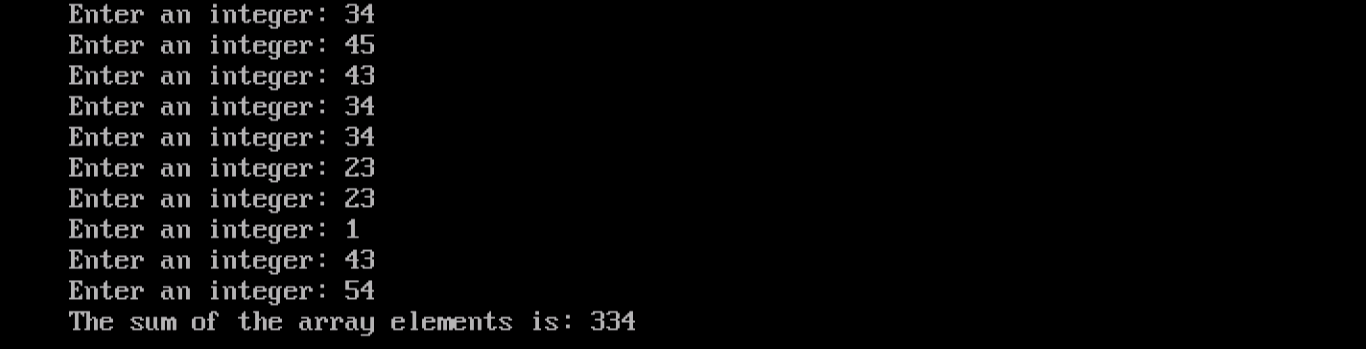
2) Write a program to find number of odd and even elements from the 1- D array.
#include<stdio.h>
#include<conio.h>
void main()
{
int i,arr[10],even=0,odd=0;
clrscr();
for(i=0;i<=9;i++){
scanf("%d",&arr[i]);
if (arr[i]%2==0){
even++;
}
else
{
odd++;
}
}
printf("The number of even elements in the array is: %d\n", even);
printf("The number of odd elements in the array is: %d\n", odd);
getch();
}

3) Write a program to reverse the elements of array and store it in another array.
#include<stdio.h>
#include<conio.h>
void main()
{
int arr[5],i;
int rev_arr[5];
clrscr();
printf("Enter Array : ");
for (i=0;i<5;i++)
{
scanf("%d",&arr[i]);
}
for (i=0;i<5;i++)
{
rev_arr[i] = arr[4 - i];
}
printf("Original array : ");
for (i=0;i<5;i++)
{
printf("%d ",arr[i]);
}
printf("\nReversed array : ");
for (i=0;i<5;i++)
{
printf("%d ",rev_arr[i]);
}
getch();
}

4) Write a program to sort elements of array.
#include<stdio.h>
#include<conio.h>
void main()
{
int i,j,arr[5];
clrscr();
for(i=0;i<5;i++){
scanf("%d",&arr[i]);
}
for (i=0;i<5;i++)
{
for (j=0;j<4-i;j++)
{
if (arr[j]>arr[j + 1])
{
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
printf("Sorted array : ");
for (i=0;i<5;i++)
{
printf("%d ", arr[i]);
}
getch();
}

5) Write a Program to print Addition of two matrices.
#include <stdio.h>
#include<conio.h>
void main()
{
int matrix1[2][2] = {{1, 2}, {3, 4}};
int matrix2[2][2] = {{5, 6}, {7, 8}},sum_matrix[2][2];
int i,j;
clrscr();
for (i=0;i<2;i++){
for (j=0;j<2;j++){
sum_matrix[i][j] = matrix1[i][j] + matrix2[i][j];
}
}
printf("Matrix 1:\n");
for (i=0;i<2;i++){
for (j=0;j<2;j++){
printf("%d ", matrix1[i][j]);
}
printf("\n");
}
printf("\nMatrix 2:\n");
for (i=0;i<2;i++){
for (j=0;j<2;j++) {
printf("%d ", matrix2[i][j]);
}
printf("\n");
}
printf("\nSum matrix:\n");
for (i=0;i<2;i++){
for (j=0;j<2;j++){
printf("%d ", sum_matrix[i][j]);
}
printf("\n");
}
getch();
}
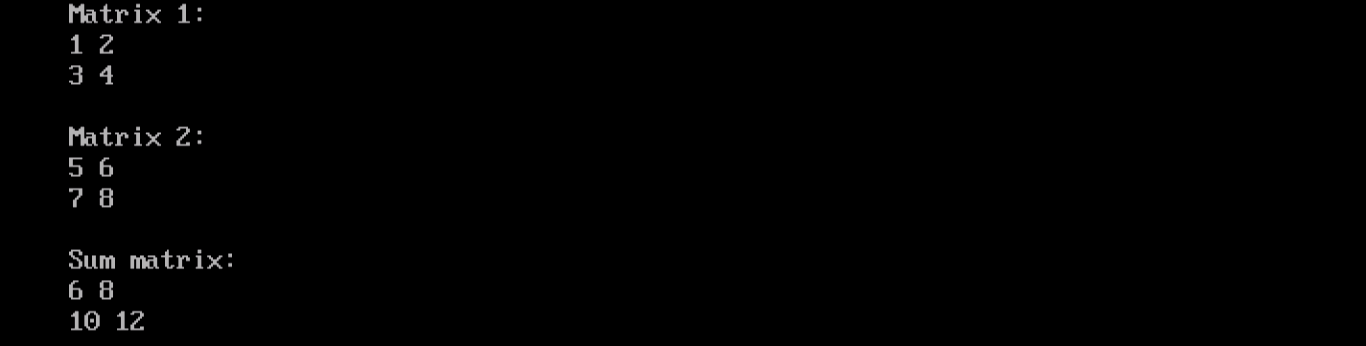
6) Program to remove duplicate numbers from a list of numbers and print the list without duplicate numbers.
#include<stdio.h>
#include<conio.h>
void main()
{
int arr[10],uq_arr[10],num_uq = 0;
int i,j;
clrscr();
printf("Enter Array : ");
for(i=0;i<10;i++){
scanf("%d",&arr[i]);
}
for (i=0;i<10;i++)
{
int is_duplicate = 0;
for (j=0;j<num_uq;j++)
{
if (arr[i] == uq_arr[j])
{
is_duplicate = 1;
break;
}
}
if (!is_duplicate)
{
uq_arr[num_uq] = arr[i];
num_uq++;
}
}
printf("List without duplicates: ");
for (i=0;i<num_uq;i++)
{
printf("%d ", uq_arr[i]);
}
getch();
}
7)Write a Program to print Multiplication of two matrices.
#include <stdio.h>
#include<conio.h>
void main()
{
int a[3][3], b[3][3], result[3][3]=0;
clrscr();
printf("Enter the elements of matrix 1:\n");
for (i=0;i<3;i++){
for (j=0;j<3;j++){
scanf("%d", &a[i][j]);
}
}
printf("Enter the elements of matrix 2:\n");
for (i=0;i<3;i++) {
for (j=0;j<3;j++){
scanf("%d", &b[i][j]);
}
}
for (i=0;i<3;i++){
for (j=0;j<3;j++)
{
result[i][j] += a[i][k] * b[k][j];
}
}
printf("Result matrix:\n");
for (i=0;i<3;i++){
for (j=0;j<3;j++){
printf("%d ", result[i][j]);
}
printf("\n");
}
getch();
}

8) Read the marks of five subjects obtained by five students in an examination. Display the top two student’s codes and their marks.
#include <stdio.h>
#include<conio.h>
void main()
{
int marks[5][5];
int std=5,sub=5,i,j;
int max1 = 0, max2 = 0, code1, code2, total_marks = 0;
clrscr();
printf("Enter the marks of %d students for %d subjects:\n", std, sub);
for (i=0;i<std;i++)
{
printf("Enter the marks for student %d:\n", i + 1);
for (j=0;j<sub;j++)
{
scanf("%d", &marks[i][j]);
}
}
for (i=0;i<std;i++)
{
for (j=0;j<sub;j++) {
total_marks += marks[i][j];
}
if (total_marks > max1)
{
max2 = max1;
code2 = code1;
max1 = total_marks;
code1 = i + 1;
}
else if (total_marks > max2)
{
max2 = total_marks;
code2 = i + 1;
}
}
printf("Top two students:\n");
printf("Code %d: %d marks\n", code1, max1);
printf("Code %d: %d marks\n", code2, max2);
getch();
}
9) Write a program to insert an element in an array at specified position.
#include <stdio.h>
#include<conio.h>
void main()
{
int arr[100],n, pos, val;
int i,j;
clrscr();
printf("Enter the size of the array (maximum 100): ");
scanf("%d", &n);
printf("Enter %d elements of the array:\n", n);
for (i=0;i<n;i++)
{
scanf("%d", &arr[i]);
}
printf("Enter the position where you want to insert the element: ");
scanf("%d", &pos);
printf("Enter the value of the element to be inserted: ");
scanf("%d", &val);
for (i=n;i>pos;i--)
{
arr[i] = arr[i - 1];
}
arr[pos] = val;
n++;
printf("The updated array is:\n");
for (i=0;i<n;i++)
{
printf("%d ", arr[i]);
}
printf("\n");
getch();
}

10) Write a program to find the length of a string.
#include <stdio.h>
#include<conio.h>
#include <string.h>
void main()
{
char str[100];
int len;
clrscr();
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
if (str[strlen(str) - 1] == '\n')
{
str[strlen(str) - 1] = '\0';
}
len = strlen(str);
printf("The length of the string is: %d\n", len);
getch();
}

11) Write a program to reverse the string.(without inbuilt Function)
#include <stdio.h>
#include<conio.h>
void main()
{
char str[100];
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
if (str[strlen(str) - 1] == '\n')
{
str[strlen(str) - 1] = '\0';
}
// Reverse the string
int i, j;
char temp;
for (i = 0, j = strlen(str) - 1; i < j; i++, j--)
{
temp = str[i];
str[i] = str[j];
str[j] = temp;
}
printf("The reversed string is: %s\n", str);
getch();
}

12) Write a program to convert a string in to lower case and upper case.
#include <stdio.h>
#include<conio.h>
#include <string.h>
void main()
{
char str[100];
int i;
clrscr();
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
if (str[strlen(str) - 1] == '\n')
{
str[strlen(str) - 1] = '\0';
}
for (i = 0; i < strlen(str); i++)
{
if (str[i] >= 'A' && str[i] <= 'Z') {
str[i] += 32;
}
}
printf("The string in lowercase is: %s\n", str);
for (i = 0; i < strlen(str); i++)
{
if (str[i] >= 'a' && str[i] <= 'z')
{
str[i] -= 32;
}
}
printf("The string in uppercase is: %s\n", str);
getch();
}

13) Write a menu driven program for the implementation of all build-in string functions.
#include <stdio.h>
#include <string.h>
#include<conio.h>
void main() {
char str[100],ch;
int choice, length, i, j, cmp;
char copy[100],str2[100],str3[100];
clrscr();
do {
printf("\nMenu:\n");
printf("1. String length\n");
printf("2. String copy\n");
printf("3. String concatenation\n");
printf("4. String comparison\n");
printf("5. String reverse\n");
printf("6. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch(choice) {
case 1:
printf("\nEnter a string: ");
scanf("%s", str);
length = strlen(str);
printf("Length of the string is %d\n", length);
break;
case 2:
printf("\nEnter a string: ");
scanf("%s", str);
strcpy(copy, str);
printf("Original string: %s\n", str);
printf("Copied string: %s\n", copy);
break;
case 3:
printf("\nEnter a string: ");
scanf("%s", str);
printf("Enter another string: ");
scanf("%s", str2);
strcat(str, str2);
printf("Concatenated string: %s\n", str);
break;
case 4:
printf("\nEnter a string: ");
scanf("%s", str);
printf("Enter another string: ");
scanf("%s", str3);
cmp = strcmp(str, str3);
if(cmp == 0) {
printf("The strings are equal\n");
} else if(cmp < 0) {
printf("The first string is less than the second string\n");
} else {
printf("The first string is greater than the second string\n");
}
break;
case 5:
printf("\nEnter a string: ");
scanf("%s", str);
length = strlen(str);
for(i = 0, j = length-1; i < j; i++, j--) {
ch = str[i];
str[i] = str[j];
str[j] = ch;
}
printf("Reversed string: %s\n", str);
break;
case 6:
printf("\nExiting the program\n");
break;
default:
printf("\nInvalid choice. Please try again.\n");
break; }
} while(choice != 6);
getch();
}

14) Program to extract n characters starting from m in a given string. (String, n and m should be provided as inputs).
#include <stdio.h>
#include<conio.h>
#include <string.h>
void main()
{
char str[100];
int n, m, i,sub_len=0;
printf("Enter a string: ");
gets(str);
printf("Enter the value of m: ");
scanf("%d", &m);
printf("Enter the value of n: ");
scanf("%d", &n);
char sub_str[100];
for (i = m; i < strlen(str) && sub_len < n; i++)
{
sub_str[sub_len++] = str[i];
}
sub_str[sub_len] = '\0';
printf("The extracted string is: %s\n",sub_str);
getch();
}

15) Find out occurrence of each character in a given string.
#include <stdio.h>
#include<conio.h>
#include <string.h>
#define MAX_CHARS 256
void main()
{
char s[100];
int count[MAX_CHARS] = {0},i;
clrscr();
printf("Enter a string: ");
gets(s);
for (i = 0; i <strlen(s); i++) {
count[s[i]]++;
}
printf("Character\tOccurrence\n");
for (i = 0; i < MAX_CHARS; i++)
{
if (count[i] > 0) {
printf("%c\t\t%d\n", i, count[i]);
}
}
getch();
}
