

Solution :
Ans 1(A)
- Arithmetic Operators
- Relational Operators.
- Logical Operators
- Assignment Operators
- increment and Decrement Operators
- Bitwise Operators
- Conditional Operators
- Special Operators
We use the ternary operator in C to run one code when the condition is true and another code when the condition is false.
The syntax of ternary operator is :
Test Condition ? expression1 : expression 2;
#include<stdio.h>
#include<conio.h>
void main() {
int age;
clrscr();
printf("Enter your age: ");
scanf("%d", &age);
(age >= 18) ? printf("You can vote") : printf("You cannot vote");
getch();
}
Ans 1(B)
In C programming, a switch statement is used to evaluate an expression against a set of predefined cases. The syntax of the switch statement is as follows:
switch (expression) { case constant1: // code to be executed if expression is equal to constant1 break; case constant2: // code to be executed if expression is equal to constant2 break; . . . default: // code to be executed if expression doesn't match any of the constants }
Here, the expression is evaluated against a set of constants defined by the cases. If the expression matches a particular constant, the code inside that case is executed. If no match is found, the code inside the default case is executed.
The switch statement is often used as an alternative to multiple if-else statements. It can make code more concise and easier to read in situations where there are multiple possible values for a variable.
A flowchart of the switch statement might look something like this :
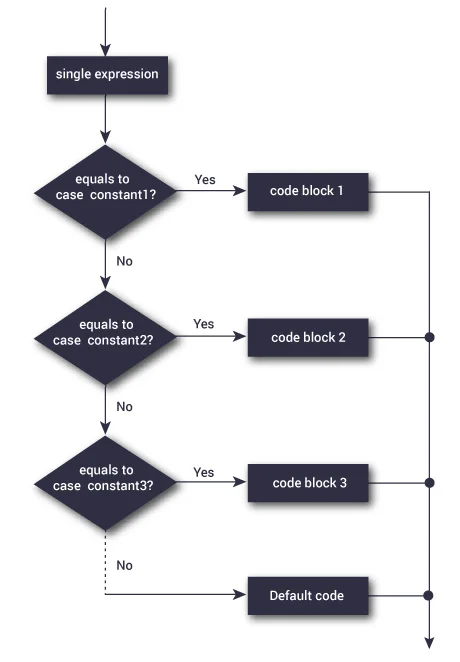